A large number of other people know in regards to the WooCommerce API however simply haven’t gotten round to the usage of it. Others are simply finding out about it. Nonetheless, other people may have tried to make use of it however were given caught someplace alongside the way in which and went again to the nice previous techniques of doing issues.
In fact, the WooCommerce API isn’t particularly difficult however – like all new method or product function – it does take a bit of being used to. One of the best ways to rise up to hurry on one thing like that is to discover a great, straight forward, easy-to-understand educational that takes you step-by-step thru a procedure – even supposing it’s now not essentially the most real-world instance. Not anything over-the-top – only a fast evidence of idea script to peer if, and the way, you’ll get it running. One thing that can can help you render a web page like this:
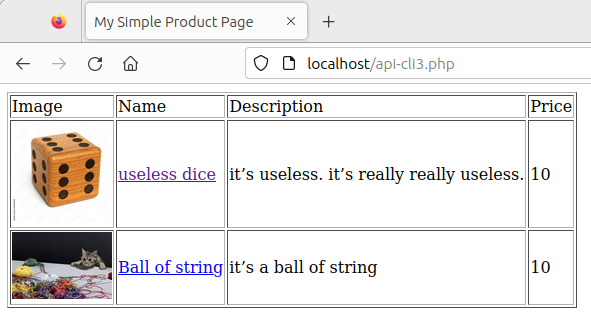
After a couple of mins’ seek, you’ll have discovered there’s a ton of excellent knowledge on such things as what the WooCommerce API is; an inventory of supported endpoints; the right way to generate an API key; and the right way to take a look at the API in a device like Postman or Insomnia.
What you gained’t to find are articles that provide an explanation for the right way to take the next move: to move from a running GET request in an API trying out device to a useful (if elementary) script that attracts knowledge from your WooCommerce set up and presentations it someplace that isn’t your WordPress web page – whether or not that’s a separate internet server or a cell app or one thing else.
That’s what this put up goes to turn you: A step by step information for your first “Hi Woo” implementation the usage of the WooCommerce API.
The pattern script I’m construction as of late is deliberately straight forward and simplistic as a result of I don’t need the main points to get misplaced in a flashy little bit of code. The instance I’m construction:
- is constructed the usage of PHP (however it will have to be straight forward to take this and turn to Node, Python, or your no matter.
- pulls an inventory of goods from an current woocommerce web page.
- presentations the picture, title, description, and worth in a plain-and-simple HTML desk.
Earlier than You Get started Coding
To stay this put up quick and readable, I’m presuming you’ve already completed a couple of steps. However there are hyperlinks – each previous on this weblog and the bullets under – to the right way to do the ones duties.
- You might have a running set up of WordPress and WooCommerce.
- You might have no less than one or two merchandise printed within the WooCommerce store.
- You’ve created a read-only API key, and you’ve got the patron key and client secret at hand.
- You’ve effectively gotten a reaction from the URL “http://YOUR_SERVER/wp-json/wc/v3/merchandise” in a device like Postman or Insomnia.
- “YOUR_SERVER” is, (optimistically) clearly, the URL of your exact WordPress set up.
- You might have a separate machine working a internet server that’s able to rendering PHP code.
As soon as that’s all in position, we’re in a position to begin coding!
Oauth for the Confused
It’s an indisputable fact that the item that journeys up new builders when they are trying their hand with the WooCommerce API is getting authentication to paintings.
This has not anything to do with WooCommerce and the entirety to do with the truth that OAuth is solely… bizarre. And difficult to wrap your head round. And infuriatingly under-documented – particularly for brand spanking new builders. For now, I’m going to give an explanation for only some issues about OAuth because it pertains to WooCommerce to get you over that hump.
- A straight forward WooCommerce API operation makes use of OAuth model 1.0.
- Now not Fundamental Auth, as some knowledge out on the net may say.
- Oauth 2.0 is NOT backward suitable with OAuth 1.0. So when you’ve got a machine that helps 2.0, you will have so as to add some libraries to get this script running.
- Explicit to PHP, there are a couple of other OAuth purchasers. For this situation, I’m the usage of the (roughly) local PHP oauth module. I’m the usage of it as it’s straight forward, now not as it’s the most efficient. For a real-world implementation, you’ll virtually surely need to use one thing more moderen and extra tough.
- Without reference to which shopper you employ, you’ll want to ensure you’ll generate the next parts:
- A timestamp
- A “nonce” – a random quantity.
- an OAuth signature
It’s adequate in case you aren’t 100% certain what these things are since you’ll see them in position within the instance under. However I sought after you to grasp those required parts prior to we were given too deep into the code.
However with all of that mentioned…
Let’s Get Coding!
I’m going to construct our script in levels so you’ll perceive the important thing parts as they layer on peak of one another.
Degree One: Get Authenticated, Get Knowledge, Get Out.
$oauth->setNonce($nonce);
$sig = $oauth->generateSignature(‘GET’,$url);
#create the CURL command
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => $url.’?oauth_consumer_key=’.$consumerKey.’&oauth_signature_method=HMAC-SHA1&oauth_timestamp=’.$timestamp.’&oauth_nonce=”.$nonce.”&oauth_version=1.0&oauth_signature=”.$sig,
CURLOPT_RETURNTRANSFER => true,
));
# execute the curl command and go back the information
$reaction = curl_exec($curl);
curl_close($curl);
echo $reaction;
?>” data-lang=”utility/x-httpd-php”>
<?php
# Set some preliminary variables
$url = "http://localhost:8888/wp-json/wc/v3/merchandise';
$consumerKey = '1234567890';
$consumerSecret="09876564321";
$nonce = mt_rand();
$timestamp = time();
#arrange oauth
$oauth = new OAuth($consumerKey,$consumerSecret);
$oauth->setTimestamp($timestamp);
$oauth->setNonce($nonce);
$sig = $oauth->generateSignature('GET',$url);
#create the CURL command
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => $url.'?oauth_consumer_key='.$consumerKey.'&oauth_signature_method=HMAC-SHA1&oauth_timestamp='.$timestamp.'&oauth_nonce=".$nonce."&oauth_version=1.0&oauth_signature=".$sig,
CURLOPT_RETURNTRANSFER => true,
));
# execute the curl command and go back the information
$reaction = curl_exec($curl);
curl_close($curl);
echo $reaction;
?>
At this level, many of the paintings taking place is the OAuth connection. A couple of issues to notice:
- I cut up out the URL, client key, and many others. into separate straight forward variables.
- The shopper key and client secret you notice above are (clearly) pretend. Please use your genuine ones.
- Similarly glaring is that you simply will have to by no means hard-code your keys into your code. I’m doing it right here for the sake of readability solely.
- There are extra sublime techniques to mix all the ones variables into the
CURLOPT_URL
. As soon as once more, I introduced them in the way in which I did to make the code smooth to practice, now not as it’s one of the best ways to do it.
If all is going smartly, you’ll run this script from the command line, and can get output that appears one thing like this:
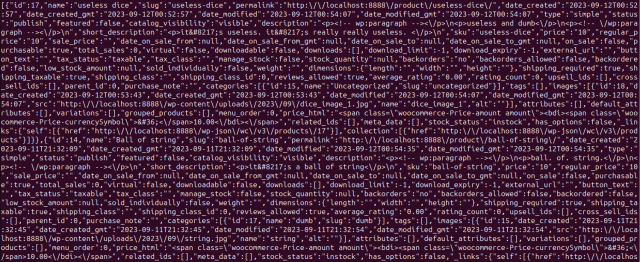
It’s now not lovely, however you’ll see that we’re pulling records from the WooCommerce web page, together with the title, description, URLs, and a number of different knowledge. And that’s just right sufficient to allow us to transfer to the next move.
Degree Two: Extracting the Knowledge We Want
<?php
# Set some initial variables
### CODE MISSING HERE ###
# execute the curl command and return the data
$curl_response = curl_exec($curl);
curl_close($curl);
$response_array = json_decode($curl_response,true);
foreach($response_array as $product) {
$name = $product['name'];
$permalink = $product['permalink'];
$short_desc = $product['short_description'];
$worth = $product['price'];
foreach($product['images'] as $symbol) {
$symbol = $symbol['src'];
ruin;
}
echo $title.', '.$permalink.', '.$short_desc.', '.$worth.', '.$symbol."n";
}
?>
The important thing side of this model is that we’re the usage of a for every loop to cycle thru every of the goods and extracting the information parts we would like: The title, description, and worth; together with the picture hyperlink and a to product at the WooCommercce server itself.
A couple of main points I’d like to name out:
- The whole thing from the road
# Set some preliminary variables
to# execute the curl command and go back the information
is equal to the former level, so I didn’t trouble to incorporate it. A complete model of every of the scripts is to be had as a obtain on the finish of this put up. - The uncooked output of our API name is in string structure, so I added
json_decode()
it so PHP can procedure the weather as an array. - there’s the potential of more than one photographs for a unmarried product. My code grabs the primary symbol and exits.
- It’s 100% most likely that the code will ruin in case your product doesn’t have a picture.
As prior to, you’ll run this script instantly from the command line, with the output having a look one thing like this:

You’ll see that we’ve were given a far clearer image of the information we’re extracting. The one level to note is that the HTML formatting within the description continues to be provide.
Having gotten thus far, our script is in a position to reach its 3rd and ultimate shape.
Degree 3: Able for Manufacturing!
“.”n”;
foreach($response_array as $product) {
$title = $product[‘name’];
$permalink = $product[‘permalink’];
$short_desc = $product[‘short_description’];
$worth = $product[‘price’];
foreach($product[‘images’] as $symbol) {
$symbol = $symbol[‘src’];
ruin;
}
$productlist = $productlist.’
‘.”n”;
}
?>
” data-lang=”utility/x-httpd-php”>
<?php
# Set some preliminary variables
### CODE (nonetheless) MISSING HERE ###
# execute the curl command and go back the information
$curl_response = curl_exec($curl);
curl_close($curl);
$response_array = json_decode($curl_response,true);
$productlist="<tr><td>Symbol</td><td>Title</td><td>Description</td><td>Worth</td></tr>"."n";
foreach($response_array as $product) {
$title = $product['name'];
$permalink = $product['permalink'];
$short_desc = $product['short_description'];
$worth = $product['price'];
foreach($product['images'] as $symbol) {
$symbol = $symbol['src'];
ruin;
}
$productlist = $productlist.'<tr><td><IMG SRC="'.$symbol.'" width="100"></td><td><A HREF="'.$permalink.'"">'.$title.'</a></td><td>'.$short_desc.'</td><td>'.$worth.'</td></tr>'."n";
}
?>
<!DOCTYPE html>
<html>
<head>
<identify>My Easy Product Web page</identify>
</head>
<frame>
<desk border=1>
<?php echo $productlist; ?>
</desk>
</frame>
</html>
On this iteration of the code, we’re simply including the weather that let it to show as a internet web page. The ones come with:
- The
$productlist
variable, that wraps the information into an HTML desk construction. - Exhibiting the product symbol quite than list the URL.
- Combining the title with the product URL in order that the title is clickable.
The outcome, as we noticed previous, will have to seem like this:
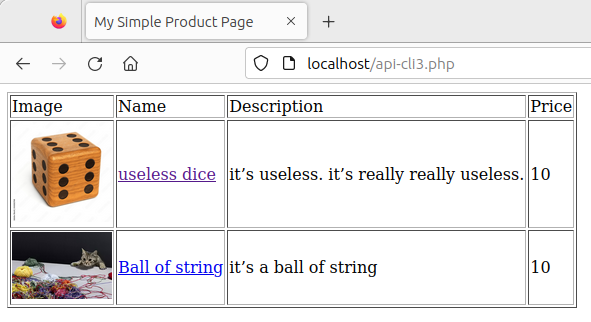
The (Most commonly) Pointless Abstract
The important thing takeaway from all that is that putting in and the usage of the WooCommerce API isn’t in particular tough. Having noticed a straight forward instance, it’s most likely you have already got concepts on the right way to prolong and strengthen what you’ve realized right here. And the most efficient phase is that now you might have the information to show the ones concepts into fact!